Java Program To Calculate Square Root Of A Number
The following computes floor(sqrt(N)) for N 0: x = 2^ceil(numbits(N)/2) loop: y = floor((x + floor(N/x))/2) if y = x return x x = y This is a version of Newton's method given in Crandall & Pomerance, 'Prime Numbers: A Computational Perspective'. The reason you should use this version is that people who know what they're doing have proven that it converges exactly to the floor of the square root, and it's simple so the probability of making an implementation error is small.
It's also fast (although it's possible to construct an even faster algorithm - but doing that correctly is much more complex). A properly implemented binary search can be faster for very small N, but there you may as well use a lookup table. To round to the nearest integer, just compute t = floor(sqrt(4N)) using the algorithm above.
Method to return square of a number. Fastest way to determine if an integer's square root is an integer. Java Inventory Program problems. The Java sqrt Function is one of the Java Math Library. In this Java program we will show you, How to find the. (double number)) to find the square root of. To find a square root, you simply need to find a number which, raised to the power of 2 (although just multiplying by itself is a lot easier programmatically;) ) gives back the input. So, start with a guess. If the product is too small, guess larger. If the new product is too large, you've narrowed it down - guess somewhere in between.
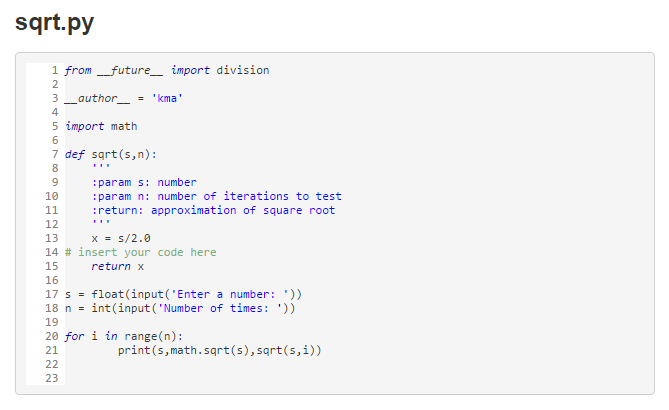
If the least significant bit of t is set, then choose x = (t+1)/2; otherwise choose t/2. Note that this rounds up on a tie; you could also round down (or round to even) by looking at whether the remainder is nonzero (i.e.
Whether t^2 4N). Note that you don't need to use floating-point arithmetic. In fact, you shouldn't.
This algorithm should be implemented entirely using integers (in particular, the floor functions just indicate that regular integer division should be used). A simple (but not very fast) method to calculate the square root of X: squareroot(x) if xErrorMargin) a = (a+b)/2 b = x/a endwhile return a; Example: squareroot(70000) a b 1 1 2 17502 4 8753 8 43 32 1116 63 590 119 355 197 276 254 265 264 As you can see it defines an upper and a lower boundary for the square root and narrows the boundary until its size is acceptable. There are more efficient methods but this one illustrates the process and is easy to understand. Just beware to set the Errormargin to 1 if using integers else you have an endless loop.
Calculate square root with arbitrary precision in Python #!/usr/bin/env python import decimal def sqrt(n): assert n 0 with decimal.localcontext as ctx: ctx.prec += 2 # increase precision to minimize round off error x, prior = decimal.Decimal(n), None while x!= prior: prior = x x = (x + n/x) / 2 # quadratic convergence return +x # round in a global context decimal.getcontext.prec = 80 # desirable precision r = sqrt(12345) print r print r decimal.Decimal(12345).sqrt Output: 1 True.
Here's something to think about: To find a square root, you simply need to find a number which, raised to the power of 2 (although just multiplying by itself is a lot easier programmatically;) ) gives back the input. So, start with a guess. If the product is too small, guess larger. If the new product is too large, you've narrowed it down - guess somewhere in between.
Calculate Square Feet
You see where I'm going. Depending on your need of precision and/or performance, there are of course lots of ways. The solution hinted at in this post is in no way the best one in either of those categories, but it gives you a clue on one way to go.